Array
Data Structure
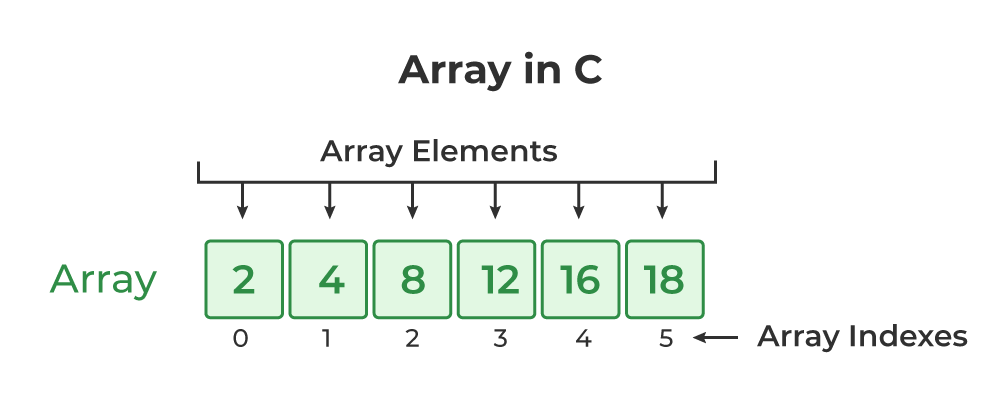
What is Array?
An array is a collection of items stored at contiguous memory locations. The idea is to store multiple items of the same type together. This makes it easier to calculate the position of each element by simply adding an offset to a base value, i.e., the memory location of the first element of the array (generally denoted by the name of the array).
For simplicity, we can think of an array as a flight of stairs where on each step is placed a value (let’s say one of your friends). Here, you can identify the location of any of your friends by simply knowing the count of the step they are on.
This makes it easier to calculate the position of each element by simply adding an offset to a base value, i.e., the memory location of the first element of the array (generally denoted by the name of the array). The base value is index 0 and the difference between the two indexes is the offset.
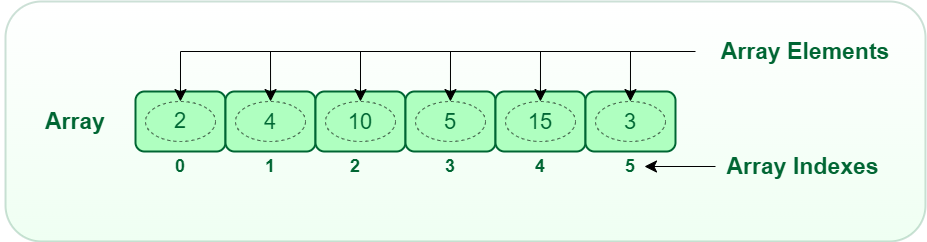
Basic terminologies of array
- Array Index: In an array, elements are identified by their indexes. Array index starts from 0.
- Array element: Elements are items stored in an array and can be accessed by their index.
- Array Length: The length of an array is determined by the number of elements it can contain.
Why Array Data Structures is needed?
Assume there is a class of five students and if we have to keep records of their marks in examination then, we can do this by declaring five variables individual and keeping track of records but what if the number of students becomes very large, it would be challenging to manipulate and maintain the data.
What it means is that, we can use normal variables (v1, v2, v3, ..) when we have a small number of objects. But if we want to store a large number of instances, it becomes difficult to manage them with normal variables. The idea of an array is to represent many instances in one variable..
Types of arrays:
There are majorly two types of arrays:
- One-dimensional array (1-D arrays): You can imagine a 1d array as a row, where elements are stored one after another.

1D array
- Two-dimensional array: 2-D Multidimensional arrays can be considered as an array of arrays or as a matrix consisting of rows and columns.
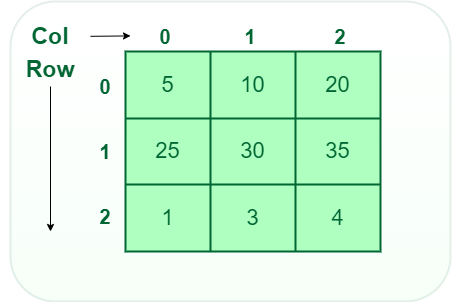
2D array
- Three-dimensional array: A 3-D Multidimensional array contains three dimensions, so it can be considered an array of two-dimensional arrays.
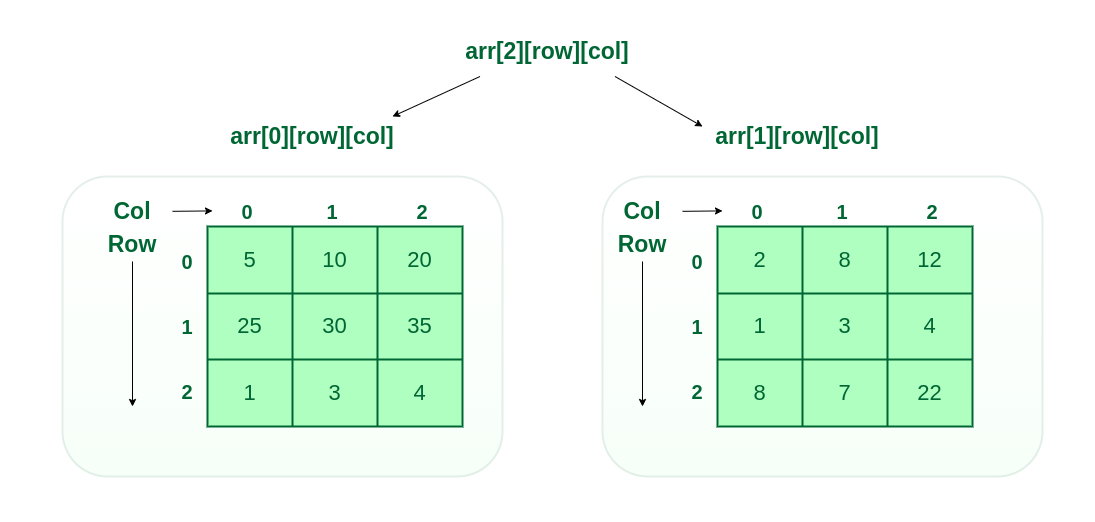
3D array
Types of Array operations:
- Traversal: Traverse through the elements of an array.
- Insertion: Inserting a new element in an array.
- Deletion: Deleting element from the array.
- Searching: Search for an element in the array.
- Sorting: Maintaining the order of elements in the array.
Advantages of using Arrays:
- Arrays allow random access to elements. This makes accessing elements by position faster.
- Arrays have better cache locality which makes a pretty big difference in performance.
- Arrays represent multiple data items of the same type using a single name.
- Arrays store multiple data of similar types with the same name.
- Array data structures are used to implement the other data structures like linked lists, stacks, queues, trees, graphs, etc.
Disadvantages of Array:
- As arrays have a fixed size, once the memory is allocated to them, it cannot be increased or decreased, making it impossible to store extra data if required. An array of fixed size is referred to as a static array.
- Allocating less memory than required to an array leads to loss of data.
An array is homogeneous in nature so, a single array cannot store values of different data types. - Arrays store data in contiguous memory locations, which makes deletion and insertion very difficult to implement. This problem is overcome by implementing linked lists, which allow elements to be accessed sequentially.
Application of Array:
- They are used in the implementation of other data structures such as array lists, heaps, hash tables, vectors, and matrices.
- Database records are usually implemented as arrays.
- It is used in lookup tables by computer.
- It is used for different sorting algorithms such as bubble sort insertion sort, merge sort, and quick sort.
Top theorotical interview Questions
S.no |
Question |
Answer |
---|---|---|
1 | What will happen if you do not initialize an Array? | View |
2 | Why is the complexity of fetching a value from an array be O(1) | View |
3 | When should you use an Array over a List? | View |
4 | What is a circularly sorted array? | View |
5 | Comparing two arrays using hashmap? | View |
6 | “What are the advantages of a linked list over an array? In which scenarios do we use LinkedList and when Array?” |
View |
7 | How do I iterate rows and columns of a multidimensional array? | View |
8 | What is meant by Sparse Array? | View |
9 | What are the benefits of Heap over Sorted Arrays? | View |
10 | Is there any difference between int[] a and int a[]? | View |
11 | Can we declare array size as a negative number? | View |
12 | We know that Arrays are objects so why cannot we write strArray.length()? | View |
13 | What are the advantages of Sorted Arrays? | View |
14 | What defines the dimensionality of an Array? | View |
15 | How to check array contains a value or not? | View |
16 | How to create an array/list inside another array/list? | View |
17 | How to get the largest and smallest number in an array? | View |
18 | How can I return coordinates/indexes of a string in a multidimensional array? | View |
19 | How do I remove objects from an array in Java? | View |
20 | How does C allocate data items in a multidimensional array? | View |
21 | Get adjacent elements in a two-dimensional array? | View |
22 | C++ How to use and pass a 3-dimensional char array? | View |
23 | Anonymous Array in Java | View |
24 | What is the default value of Array in Java? | View |
25 | How to copy an array into another array? | View |
26 | How to iterate an array in java? | View |
27 | How to merge two sorted Arrays into a Sorted Array? | View |
28 | Can we make the array volatile in Java? | View |
29 | What is the logic to reverse the array? | View |
30 | How to get the index of an array element? | View |
31 | Can we extend an array after initialization? | View |
32 | How to fill elements (initialize at once) in an array? | View |
33 | Difference between Array and String in Java | View |
34 | Print all subarrays with 0 sum | View |
35 | Equilibrium index of an array | View |
36 | How to check array contains a value or not? | View |
37 | How to get the top two numbers from an array? | View |
38 | How to implement 3 Stacks with one Array? | View |